使用Guzzle执行HTTP请求
原创文章 作者:月光光 2018年12月15日 10:18helloweba.net 标签:PHP
Guzzle是一个PHP的HTTP客户端,用来轻而易举地发送请求,并集成到我们的WEB服务上。Guzzle提供了简单的接口,构建查询语句、POST请求、分流上传下载大文件、使用HTTP cookies、上传JSON数据等等。
安装
使用Composer安装:
composer require guzzlehttp/guzzle
或者编辑项目的composer.json文件,添加Guzzle作为依赖:
{
"require": {
"guzzlehttp/guzzle": "~6.0"
}
}
然后执行composer update
Guzzle基本使用
发送请求
use GuzzleHttp\Client;
$client = new Client([
// Base URI is used with relative requests
'base_uri' => 'http://httpbin.org',
// You can set any number of default request options.
'timeout' => 2.0,
]);
$response = $client->get('http://httpbin.org/get');
$response = $client->delete('http://httpbin.org/delete');
$response = $client->head('http://httpbin.org/get');
$response = $client->options('http://httpbin.org/get');
$response = $client->patch('http://httpbin.org/patch');
$response = $client->post('http://httpbin.org/post');
$response = $client->put('http://httpbin.org/put');
设置查询字符串
$response = $client->request('GET', 'http://httpbin.org?foo=bar');
或使用query
请求参数来声明查询字符串参数:
$client->request('GET', 'http://httpbin.org', [
'query' => ['foo' => 'bar']
]);
使用响应
获取状态码:
$code = $response->getStatusCode(); // 200
$reason = $response->getReasonPhrase(); // OK
判断头部信息:
if ($response->hasHeader('Content-Length')) {
echo "It exists";
}
获取返回的头部信息:
echo $response->getHeader('Content-Length');
// Get all of the response headers.
foreach ($response->getHeaders() as $name => $values) {
echo $name . ': ' . implode(', ', $values) . "\r\n";
}
使用getBody
方法可以获取响应的主体部分(body),主体可以当成一个字符串或流对象使用
$body = $response->getBody();
可以将返回体转换成字符串或者直接以字符串形式读取:
$stringBody = (string) $body;
$content = $body->getContents();
上传文件
有时我们需要将文件传送到另一个web服务上去,可以使用post文件流形式将文件数据传送到指定web目录。
$filename = 'a.jpg';
$data = fopen($filename, 'r');
$res = $client->request('POST', 'http://localhost:9999/upload.php', ['body' => $data]);
$body = $res->getBody();
print_r($body->getContents());
接收上传文件的upload.php可以这样写:
if ($_SERVER['REQUEST_METHOD'] == 'POST') {
$data = file_get_contents('php://input');
$file = file_put_contents('b.jpg', $data);
if (FALSE === $file) {
echo '上传成功';
} else {
echo '上传失败';
}
}
提交表单
发送application/x-www-form-urlencoded
POST请求需要你传入form_params
数组参数,数组内指定POST的字段。
$res = $client->request('POST', 'http://localhost:9999/form.php', [
'form_params' => [
'field_name' => 'abc',
'other_field' => '123',
'nested_field' => [
'nested' => 'hello'
]
]
]);
$body = $res->getBody();
print_r((string)$body);
在接收端form.php使用$_POST
即可获取上传的表单数据。
提交JSON数据
有时候我们在于API接口交互的时候需要将数据以特定的json格式传给api,可以这样写:
$res = $client->request('POST', 'http://localhost:9999/json.php', [
'json' => ['foo' => 'bar']
]);
$body = $res->getBody();
print_r((string)$body);
接收端json.php使用file_get_contents('php://input')
可获得提交的json数据。
使用Guzzle还可以发送异步请求以及并发请求,具体使用方法可参照Guzzle官方文档。
其实我们在一些特殊场景下可以使用Swoole的协程特性实现异步的http客户端,功能非常强大。
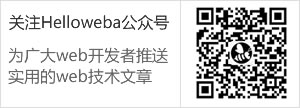
共0条评论